useDebugValue
is a React Hook that lets you add a label to a custom Hook in React DevTools.
useDebugValue
는 React 개발자 도구에서 커스텀 훅에 레이블을 추가해주는 React 훅입니다.
useDebugValue(value, format?)
Reference참조
useDebugValue(value, format?)
Call useDebugValue
at the top level of your custom Hook to display a readable debug value:
읽을 수 있는 디버그 값을 표시하려면 커스텀 훅의 최상위 레벨에서 useDebugValue
을 호출합니다.
import { useDebugValue } from 'react';
function useOnlineStatus() {
// ...
useDebugValue(isOnline ? 'Online' : 'Offline');
// ...
}
See more examples below. 아래에서 더 많은 예시를 확인하세요.
Parameters매개변수
-
value
: The value you want to display in React DevTools. It can have any type.value
: React 개발자 도구에서 표시하려는 값입니다. 모든 유형을 가질 수 있습니다. -
optional
format
: A formatting function. When the component is inspected, React DevTools will call the formatting function with thevalue
as the argument, and then display the returned formatted value (which may have any type). If you don’t specify the formatting function, the originalvalue
itself will be displayed. 선택적format
: 포매팅 함수. 컴포넌트가 검사할 때, React 개발자 도구는 인수로 포매팅 함수를 호출한 다음 반환된 포매팅된 값(모든 유형을 가질 수 있음)을 표시합니다. 포매팅 함수를 지정하지 않으면, 원본value
자체가 표시됩니다.
Returns반환값
useDebugValue
does not return anything.
useDebugValue
는 아무것도 반환하지 않습니다.
Usage사용법
Adding a label to a custom Hook 커스텀 훅에 레이블 추가
Call useDebugValue
at the top level of your custom Hook to display a readable debug value for React DevTools.
커스텀 훅의 최상위 레벨에서 useDebugValue
를 호출하여 React 개발자 도구가 읽을 수 있는 디버그 값을 표시합니다.
import { useDebugValue } from 'react';
function useOnlineStatus() {
// ...
useDebugValue(isOnline ? 'Online' : 'Offline');
// ...
}
This gives components calling useOnlineStatus
a label like OnlineStatus: "Online"
when you inspect them:
이렇게 하면 검사할 때 컴포넌트는 useOnlineStatus
를 OnlineStatus: "Online"
같은 레이블을 호출합니다.
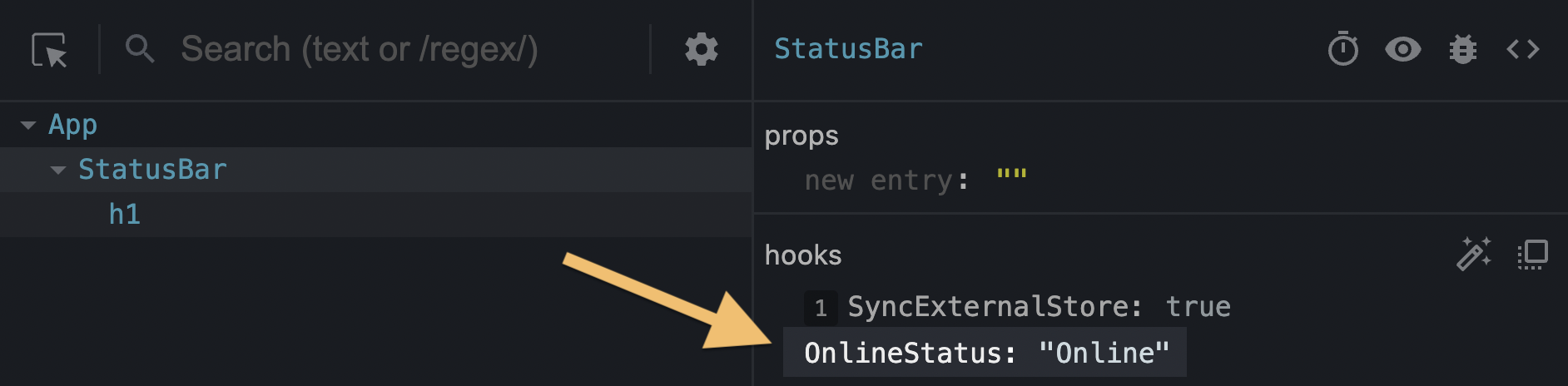
Without the useDebugValue
call, only the underlying data (in this example, true
) would be displayed.
useDebugValue
호출이 없으면 기본 데이터(예시에서는 true
)만 표시됩니다.
import { useSyncExternalStore, useDebugValue } from 'react'; export function useOnlineStatus() { const isOnline = useSyncExternalStore(subscribe, () => navigator.onLine, () => true); useDebugValue(isOnline ? 'Online' : 'Offline'); return isOnline; } function subscribe(callback) { window.addEventListener('online', callback); window.addEventListener('offline', callback); return () => { window.removeEventListener('online', callback); window.removeEventListener('offline', callback); }; }
Deferring formatting of a debug value 디버그 값의 형식 지정 연기
You can also pass a formatting function as the second argument to useDebugValue
:
useDebugValue
의 두번째 인수에 포매팅 함수를 전달할 수도 있습니다.
useDebugValue(date, date => date.toDateString());
Your formatting function will receive the debug value as a parameter and should return a formatted display value. When your component is inspected, React DevTools will call this function and display its result. 포매팅 함수는 디버그 값을 매개변수로 받고 변환된 값을 반환해야 합니다. 컴포넌트가 검사할 때, React 개발자 도구가 이 함수를 호출하고 그 결과를 표시합니다.
This lets you avoid running potentially expensive formatting logic unless the component is actually inspected. For example, if date
is a Date value, this avoids calling toDateString()
on it for every render.
이렇게 하면 컴포넌트를 실제로 조사하지 않는 한 비용이 많이 들 수 있는 포매팅 로직을 실행하지 않아도 됩니다. 예를 들어, date
가 날짜 값인 경우, 컴포넌트를 렌더링 할 때마다 toDateString()
을 호출하지 않습니다.